What is Vite.js?
In simple words, it’s development tool which you can split into two major modes:
developmentproduction
In development mode it uses local server for serve ES modules with hot replacement which updating modules during the execution of the application.
In production it uses bundled version of assets. For more detailed explanation, read what is Vite.js
Template
Template has a following simple structure:
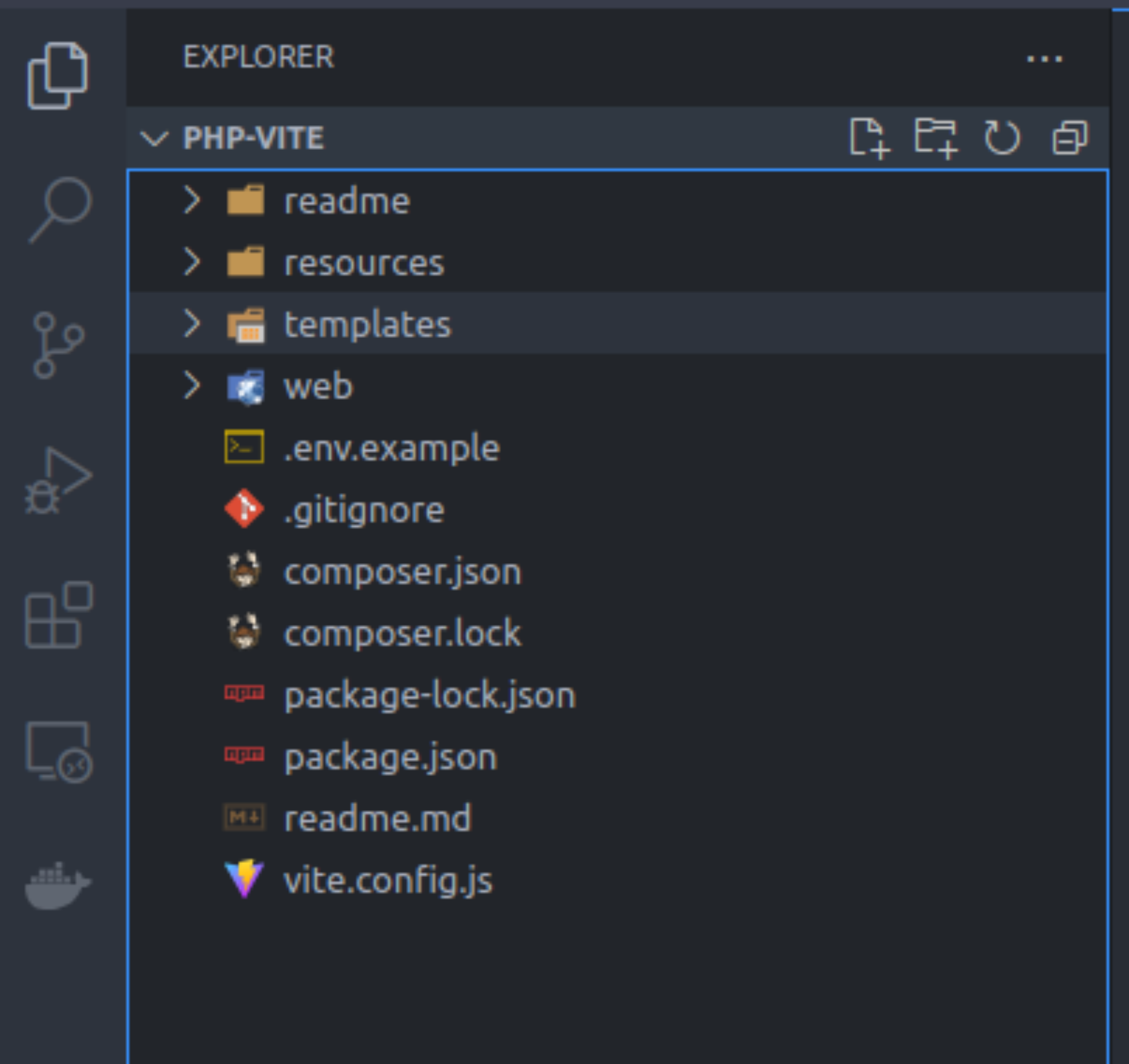
How is it working?
Take a look at the vite.config.js
import { defineConfig, loadEnv } from "vite";
import vue from '@vitejs/plugin-vue'
import liveReload from 'vite-plugin-live-reload';
import dotenv from 'dotenv';
import fs from 'fs';
// https://vitejs.dev/config/
export default ({ mode }) => {
Object.assign(process.env, dotenv.parse(fs.readFileSync(`${__dirname}/.env`)));
const port = process.env.VITE_PORT;
const origin = `${process.env.VITE_ORIGIN}:${port}`;
return defineConfig({
plugins: [
// register vue plugin
vue(),
// register live reload plugin, for refreshing the browser on file changes
liveReload([
__dirname + '/templates/**/*.php',
]),
],
base: './',
// config for the build
build: {
manifest: "manifest.json",
outDir: 'web/resources/',
rollupOptions: {
input: {
js: './resources/js/app.js',
css: './resources/scss/app.scss',
},
output: {
entryFileNames: `js/app.js`,
/* assetFileNames: `[ext]/app.[ext]`, */
assetFileNames: ({name}) => {
if (/\.(gif|jpe?g|png|svg)$/.test(name ?? '')){
return 'images/[name].[ext]';
}
if (/\.css$/.test(name ?? '')) {
return 'css/app.[ext]';
}
return '[name].[ext]';
},
},
},
},
// config for the dev server
server: {
// force to use the port from the .env file
strictPort: true,
port: port,
// define source of the images
origin: origin,
hmr: {
host: 'localhost',
},
},
resolve: {
alias: {
vue: 'vue/dist/vue.esm-bundler.js'
}
}
})
}
In simple words, this config registers plugin for Vue which we need to read Vue SFC files (.vue) and LiveReload for refreshing the browser on file changes. As you can see there is also build and server part. First one is used when we are runing “npm run build” , while server is used after “npm run dev” command. Development server is listening on host and port we registered in .env file. For example: http://localhost:5173
In webroot folder you can find index.php which is entry point of our application from the web.
use Dotenv\Dotenv;
define('BASE_PATH', dirname(__DIR__));
define('VENDOR_PATH', BASE_PATH.'/vendor');
// Load Composer's autoloader
require_once VENDOR_PATH.'/autoload.php';
if (class_exists('Dotenv\Dotenv') && file_exists(BASE_PATH . '/.env')) {
$dotenv = Dotenv::createImmutable(BASE_PATH);
$dotenv->load();
}
// Run the application!
include BASE_PATH.'/templates/page.php';
It loads composer dependencies and .env file. Then it runs application.
In page.php we need to imports Vite config inside head tags:
<?php $isDev = isset($_ENV['ENVIRONMENT']) && $_ENV['ENVIRONMENT'] === 'dev' && isset($_ENV['VITE_PORT']) ?>
<?php if($isDev) : ?>
<script type="module" src="<?php echo $_ENV['VITE_ORIGIN'] ?>:<?php echo $_ENV['VITE_PORT'] ?>/@vite/client"></script>
<script type="module" src="<?php echo $_ENV['VITE_ORIGIN'] ?>:<?php echo $_ENV['VITE_PORT'] ?>/resources/js/app.js"></script>
<script type="module" src="<?php echo $_ENV['VITE_ORIGIN'] ?>:<?php echo $_ENV['VITE_PORT'] ?>/resources/scss/app.scss"></script>
<?php else: ?>
<script type="module" src="/resources/js/app.js"></script>
<link rel="stylesheet" href="/resources/css/app.css">
<?php endif; ?>
Summary
I showed you how you can simply implement PHP project with Vite and Vue. Thanks for reading!